Macro
is the powerful feature of the laravel framework. Macros allow you to add on custom functionality to internal Laravel components like response:: macro, laravel macroable etc. This laravel macro also work with laravel apps.
Laravel Macro
Example 1
Let’s say you are working with the api and want the below response on success
{
"success": true,
"msg": "Your message",
"data": Array()
}
To achieve the above response. Open the AppServiceProvider.php file and put the below code
Request::macro(‘success’, function ($msg, $data) {
Request::json([
"success" => true,
"msg" => $msg,
"data" => $data
]);
});
And then use the above defined method
Request::success('Your message', Array());
Example 2
Let’s see another example. Suppose you want to create a new method which we will use at the time of fail
Open the AppServiceProvider.php
file and put the below code
Request::macro(‘fail’, function ($msg, $data) {
Request::json([
"success" => false,
"msg" => $msg,
]);
});
And then use
Request::fail('Your message');
Will return
{
"success": false,
"msg": "Your message"
}
You can create another macro according to your requirement using laravel macro
Which components are “Macroable” in laravel?
Macros can be defined on any class with the Macroable
trait. Below is a list of Macroable facades & classes:
Facades
- Cache
- File
- Lang
- Request
- Response
- Route
- URL
Illuminate Classes
- Illuminate\Routing\UrlGenerator
- Illuminate\Cache\Repository
- Illuminate\Validation\Rule
- Illuminate\Console\Scheduling\Event
- Illuminate\Database\Eloquent\Builder
- Illuminate\Database\Eloquent\Relation
- Illuminate\Database\Query\Builder
- Illuminate\Filesystem\Filesystem
- Illuminate\Foundation\Testing\TestResponse
- Illuminate\Http\RedirectResponse
- Illuminate\Http\Request
- Illuminate\Http\UploadedFile
- Illuminate\Routing\ResponseFactory
- Illuminate\Routing\Router
- Illuminate\Support\Str
- Illuminate\Support\Arr
- Illuminate\Translation\Translator
- Illuminate\Support\Collection
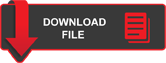