I will explain how to send email from localhost in PHP, So Email functionality is very important almost every web based application, web project, so web developers checked the emailfunctionality on local server like XAMPP, WAMP or any other local server before uploading email functionality on the live server.
There are many way you can send the email in php by using PHPMailer and php mail()function but in this article, i will show you how to send email from localhost in php by using PHPMailer. You can use any local server like, XAMPP, WAMP etc., but i am using XAMPPserver as a localhost server.
In this article need followings.
- PHPMailer File
- email.php(HTML Form)
- sendemail.php(PHP Code), also
- SMTP server of any of your mail accounts like gmail, yahoo, outlook, hotmail, etc.(but in this article, i am using gmail SMTP server)
Follows These Steps
1. Download PHPMailer
Go to browser and type phpmailer, you can download from any link but, I will provide one of the link https://github.com/PHPMailer/PHPMailer screen shown below
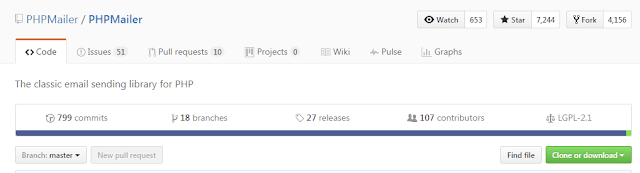
Now click Clone or download after clicked you can see two options one is Open in Desktopand another one is Download ZIP, screen shown below
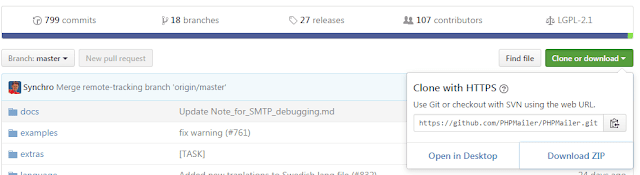
then you can click Download ZIP
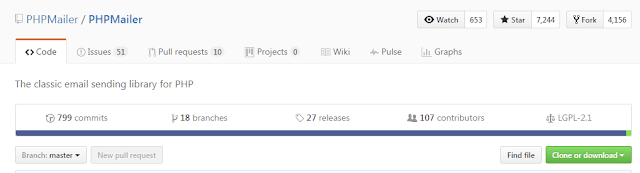
Now click Clone or download after clicked you can see two options one is Open in Desktopand another one is Download ZIP, screen shown below
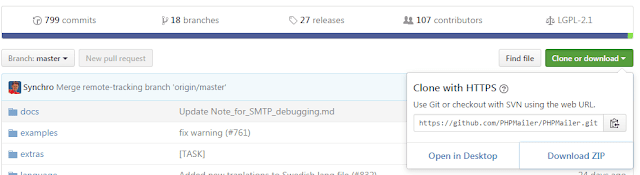
then you can click Download ZIP
Now go to downloaded file(PHPMailer-master) and extract that file, copy the extracted file and paste in project root folder for example my project root folder is tutorials, path like C:\xampp\htdocs\tutorials\PHPMailer-master
2. Create email.php File
<!DOCTYPE html>
<html>
<head>
<title>Sending Email using PHP</title>
</head>
<body>
<fieldset style="width: 300px;">
<legend>Contact Us</legend>
<form action=" sendemail.php" method="post">
<table border="0" cellspacing="5" cellpadding="5">
<tr>
<td>Email To:</td>
<td> <input type="text" name="mailto" id="mailto" required /></td>
</tr>
<tr>
<td>Subject:</td>
<td><input type="text" name="subject" id="subject" required /></td>
</tr>
<tr>
<td>Message:</td>
<td><textarea id="message" name="message" rows="4" style="width: 166px;" required></textarea></td>
</tr>
<tr>
<td></td>
<td><input type="submit" name="Submit" value="Send"></td>
</tr>
</table>
</form>
</fieldset>
</body>
</html>
3. Create sendemail.php File
<?php
include 'PHPMailer-master/PHPMailerAutoload.php';
$mail = new PHPMailer;
$mail->isSMTP(); // Set mailer to use SMTP
$mail->Host = 'smtp.gmail.com'; // Specify main and backup SMTP servers
$mail->SMTPAuth = true; // Enable SMTP authentication
$mail->Username = 'XYZ@gmail.com'; // SMTP username
$mail->Password = '*******'; // SMTP password
$mail->SMTPSecure = 'ssl'; // Enable TLS encryption, `ssl` also accepted
$mail->Port = 465; // TCP port to connect to
$mail->setFrom('XYZ@gmail.com', 'gowebtuts');
$mail->addReplyTo('XYZ@gmail.com', 'gowebtuts');
$mail->addAddress('XYZ@gmail.com'); // Add a recipient
$mail->addCC('cc@gmail.com'); //Add cc Address
$mail->addBCC('bcc@gmail.com'); //Add bcc Address
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = $_POST['subject'];
$mail->Body = $_POST['message'];
$mail->addAddress($_POST['mailto']); // Add a recipient
if(!$mail->send())
{
echo 'Message could not be sent.';
echo 'Mailer Error: ' . $mail->ErrorInfo;
}
else
{
echo 'Message has been sent';
}
?>
Here,
- Include PHPMailer-master/PHPMailerAutoload.phpat
- Username section put the correct gmail id
- Password section put the correct password
- Put correct email id at XYZ
- Put correct cc and bcc address.
- SMTPSecure section put ssl and port section put 465 also you can change SMTPSecure Section tls but make sure also changed in port section put 587.
Now go to browser and type http://localhost/tutorials/mail.php, screen shown below
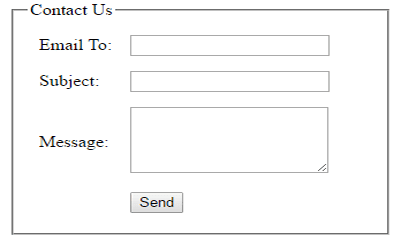
then put value in the required fields, screen shown below
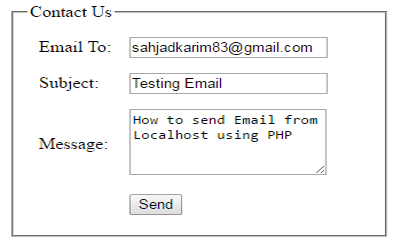
Now click Send button, screen shown below

Now just check email is sended or not, login gmail account and check email, screen shown below
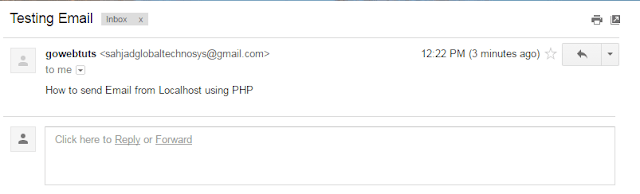
So Email is Successfully Send.
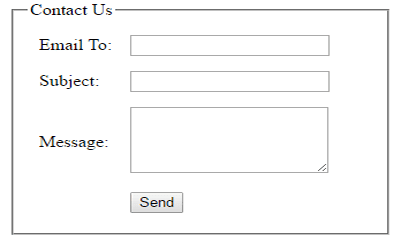
then put value in the required fields, screen shown below
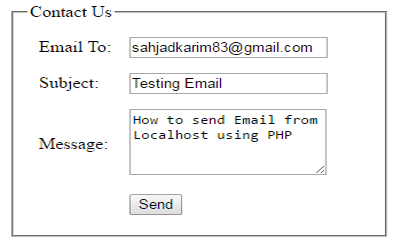
Now click Send button, screen shown below

Now just check email is sended or not, login gmail account and check email, screen shown below
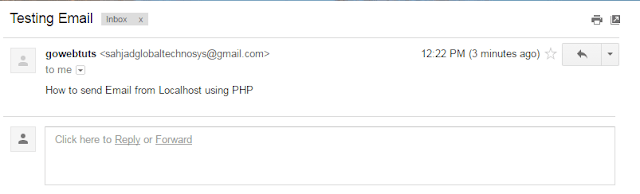
So Email is Successfully Send.
I hope, it helped you to understand, How to send email from localhost in PHP......Thanks