This Article show you how to create a simple Web Service.
Soap Server
SimpleServer.php
<?php
// Simple Method get 1 parameter and return with Hello
function AddHello($name)
{
return "Hello $name";
}
// Create SoapServer object using WSDL file.
// For the simplicity, our SoapServer is set to operate in non-WSDL mode. So we do not need a WSDL file
$server = new SoapServer(null, array('uri'=>'http://localhost/hello'));
// Add AddHello() function to the SoapServer using addFunction().
$server->addFunction("AddHello");
// To process the request, call handle() method of SoapServer.
$server->handle();
?>
Soap Client allows you to communicate with server
SimpleClient.php
<?php
include "nusoap.php"; //Soap Library.
try {
// Create a soap client using SoapClient class
// Set the first parameter as null, because we are operating in non-WSDL mode.
// Pass array containing url and uri of the soap server as second parameter.
$client = new soapclient(null, array(
'location' => "http://localhost/hello/HelloServer.php",
'uri' => "http://localhost/hello"));
// Read request parameter
$param = $_POST['name'];
// Invoke AddHello() method of the soap server (HelloServer)
$result = $client->AddHello($param);
echo $result; // Process the the result
}
catch(SoapFault $ex) {
$ex->getMessage();
}
?>
Soap View to interact for end-user
SimpleView.php
<?php
echo "<h2>Welcome to PHP Web Service</h2>";
echo "<form action='SimpleClient.php' method='POST'/>";
echo "<input name='name' /><br/>";
echo "<input type='Submit' name='submit' value='Send'/>";
echo "</form>";
?>
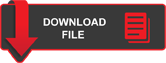