I received lots of tutorial requests from my readers in that most of them asked me, how to use Ionic 5 to create a welcome page with login and signup pages. Ionic updated there code base with latest Angular 8 features. Now we can implement the routes and guards pretty easy way. Ionic is recommending to use Capacitor to generate iOS and Android. This post will explain to you how to design an Ionic project structure with social project related pages like messages, feed, notifications, etc. Finally converting this web Ionic project to iOS and Android applications.
Prerequisites
You need to have the following software installed:
- Node 12+
- Ionic 5+
Create Ionic Project
Generate an Ionic project with tabs feature.
Run the Project
Project server will run at 8100 port.
Working with New Project Structure
Create folders like Pages, Components, and Services.
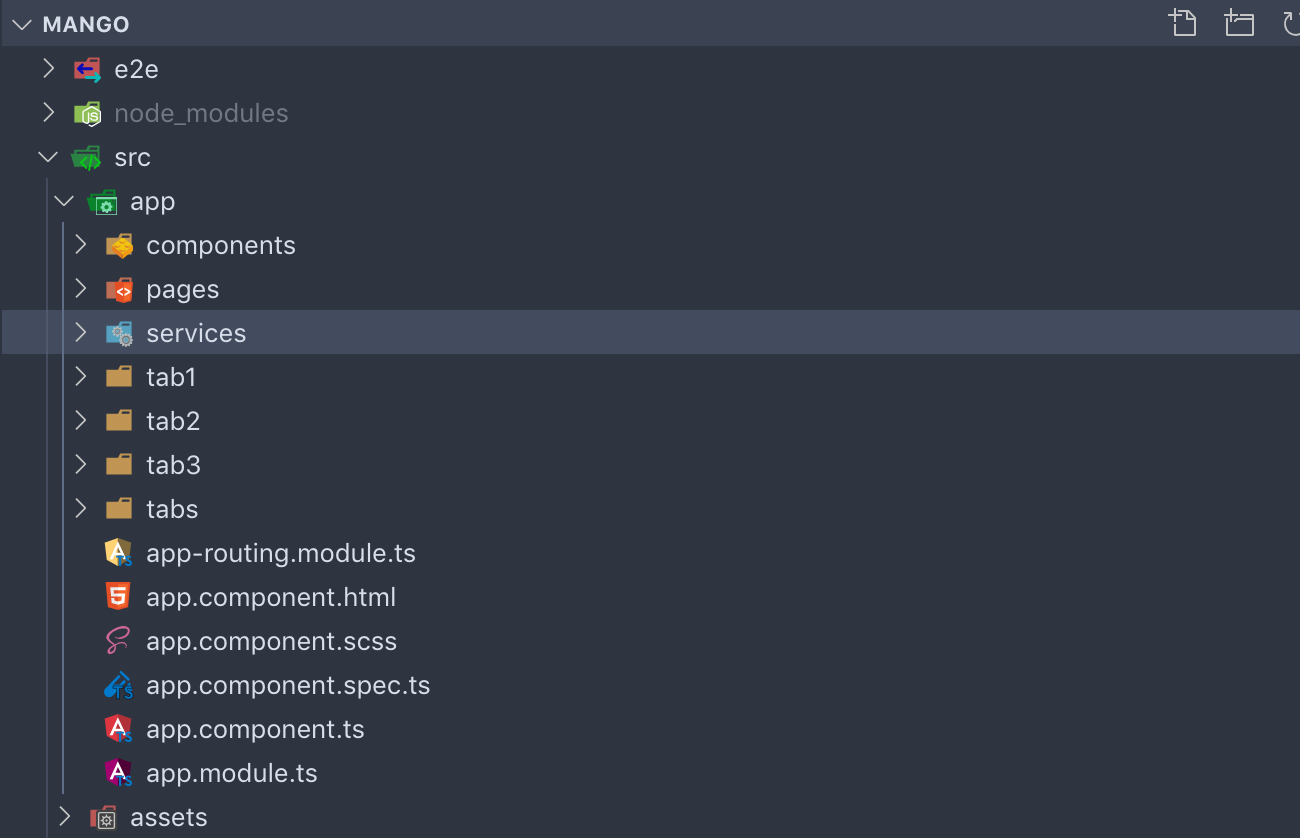
Home Container
All of the authenticated pages under this container routes. For more inforamtion read Angular Routing using Route Guards
> ng generate page home
CREATE src/app/home/home.module.ts (533 bytes)
CREATE src/app/home/home.page.scss (0 bytes)
CREATE src/app/home/home.page.html (123 bytes)
CREATE src/app/home/home.page.spec.ts (677 bytes)
CREATE src/app/home/home.page.ts (248 bytes)
UPDATE src/app/app-routing.module.ts (488 bytes)
[OK] Generated page!
Index Container
Unauthorized pages under this component.
> ng generate page index
CREATE src/app/index/index.module.ts (538 bytes)
CREATE src/app/index/index.page.scss (0 bytes)
CREATE src/app/index/index.page.html (124 bytes)
CREATE src/app/index/index.page.spec.ts (684 bytes)
CREATE src/app/index/index.page.ts (252 bytes)
UPDATE src/app/app-routing.module.ts (563 bytes)
[OK] Generated page!
Generate Unauthenticated Pages
Use Ionic command to generate unauthenticated pages like login, signup, welcome and forgot under the pages directory.
> ng generate page pages/welcome
CREATE src/app/pages/welcome/welcome.module.ts (548 bytes)
CREATE src/app/pages/welcome/welcome.page.scss (0 bytes)
CREATE src/app/pages/welcome/welcome.page.html (126 bytes)
CREATE src/app/pages/welcome/welcome.page.spec.ts (698 bytes)
CREATE src/app/pages/welcome/welcome.page.ts (260 bytes)
UPDATE src/app/app-routing.module.ts (652 bytes)
[OK] Generated page!
mango$ ionic generate page pages/login
> ng generate page pages/login
CREATE src/app/pages/login/login.module.ts (538 bytes)
CREATE src/app/pages/login/login.page.scss (0 bytes)
CREATE src/app/pages/login/login.page.html (124 bytes)
CREATE src/app/pages/login/login.page.spec.ts (684 bytes)
CREATE src/app/pages/login/login.page.ts (252 bytes)
UPDATE src/app/app-routing.module.ts (733 bytes)
[OK] Generated page!
mango$ ionic generate page pages/signup
> ng generate page pages/signup
CREATE src/app/pages/signup/signup.module.ts (543 bytes)
CREATE src/app/pages/signup/signup.page.scss (0 bytes)
CREATE src/app/pages/signup/signup.page.html (125 bytes)
CREATE src/app/pages/signup/signup.page.spec.ts (691 bytes)
CREATE src/app/pages/signup/signup.page.ts (256 bytes)
UPDATE src/app/app-routing.module.ts (818 bytes)
[OK] Generated page!
Generate Authenticated Pages
Same way generate all of the authenticated pages like feed, messages, notifications and setttings. Later we are going to apply route guards to protect these with the user authentication.
> ng generate page pages/feed
CREATE src/app/pages/feed/feed.module.ts (533 bytes)
CREATE src/app/pages/feed/feed.page.scss (0 bytes)
CREATE src/app/pages/feed/feed.page.html (123 bytes)
CREATE src/app/pages/feed/feed.page.spec.ts (677 bytes)
CREATE src/app/pages/feed/feed.page.ts (248 bytes)
UPDATE src/app/app-routing.module.ts (895 bytes)
[OK] Generated page!
mango$ ionic generate page pages/messages
> ng generate page pages/messages
CREATE src/app/pages/messages/messages.module.ts (553 bytes)
CREATE src/app/pages/messages/messages.page.scss (0 bytes)
CREATE src/app/pages/messages/messages.page.html (127 bytes)
CREATE src/app/pages/messages/messages.page.spec.ts (705 bytes)
CREATE src/app/pages/messages/messages.page.ts (264 bytes)
UPDATE src/app/app-routing.module.ts (988 bytes)
[OK] Generated page!
mango$ ionic generate page pages/notifications
> ng generate page pages/notifications
CREATE src/app/pages/notifications/notifications.module.ts (578 bytes)
CREATE src/app/pages/notifications/notifications.page.scss (0 bytes)
CREATE src/app/pages/notifications/notifications.page.html (132 bytes)
CREATE src/app/pages/notifications/notifications.page.spec.ts (740 bytes)
CREATE src/app/pages/notifications/notifications.page.ts (284 bytes)
UPDATE src/app/app-routing.module.ts (1101 bytes)
[OK] Generated page!
mango$ ionic generate page pages/settings
> ng generate page pages/settings
CREATE src/app/pages/settings/settings.module.ts (553 bytes)
CREATE src/app/pages/settings/settings.page.scss (0 bytes)
CREATE src/app/pages/settings/settings.page.html (127 bytes)
CREATE src/app/pages/settings/settings.page.spec.ts (705 bytes)
CREATE src/app/pages/settings/settings.page.ts (264 bytes)
UPDATE src/app/app-routing.module.ts (1194 bytes)
[OK] Generated page!
Lazy Routing & Navigation
You have to connect all of the pages with Index and Home containers.
index.router.ts
Create a router class and import unauthenticated pages here.
home.router.ts
Here import the user authenticated pages.
index.module.ts
Now remove the existing routes and import the IndexRouter.
home.module.ts
Follow the same and import HomeRouter.